Coroutines in Kotlin
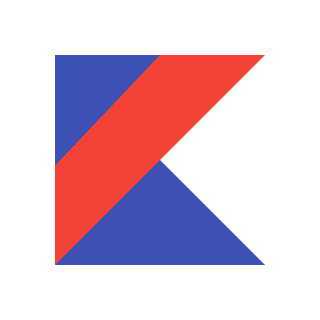
Overview Dependencies used to use Coroutines in IntelliJ Community Edition import org.jetbrains.kotlin.gradle.tasks.KotlinCompile plugins { // For build.gradle.kts (Kotlin DSL) kotlin ( "jvm" ) version "1.6.0" } group = "me.iamra" version = "1.0-SNAPSHOT" repositories { mavenCentral() } dependencies { testImplementation ( kotlin ( "test" )) implementation ( "org.jetbrains.kotlinx:kotlinx-coroutines-core:1.6.1" ) } tasks . test { useJUnit() } tasks . withType <KotlinCompile> () { kotlinOptions . jvmTarget = "1.8" } Do's and Don'ts Reference https://stackoverflow.com/questions/52522282/using-kotlinx-coroutines-in-intellij-idea-project https://github.com/Kotlin/kotlinx.coroutines/blob/master/README.md